JMock and Threads: Synchronising Access to the Mockery
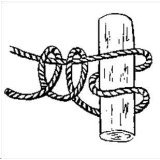
jMock's deterministic executor and scheduler help you unit-test objects that need to start or schedule concurrent tasks. They encourage the separation of system-wide threading policies from an object's essential functionality. They make unit testing easier because they run background tasks on the test thread. But, that doesn't mean that you can get away without testing the synchronization.
By default, jMock is not thread safe and so its mock objects should not be called from multiple threads. However, people frequently do use it this way. They may want to use mock objects when stress-testing the synchronisation of an object they've written and tested using the deterministic executor. Or they may want to test a class that they are implementing with a third-party library that calls into their code from multiple threads. Therefore, I've recently committed support for multithreaded tests to the JMock repository.
I've recently added a new API to jMock, the ThreadingPolicy, that the Mockery uses to synchronise access to its state. A ThreadingPolicy wraps a decorator around every Mock Object before it is imposterised and so can intercept all mocked calls entering the Mockery and all results and exceptions that it returns. The default ThreadingPolicy complains if the mock objects are used by more than one thread. But if you want to test multithreaded code you can plug a Synchroniser into the Mockery instead.
You use the Synchroniser like this:
Mockery mockery = new JUnit4Mockery() {{ setThreadingPolicy(new Synchroniser()); }};
Now any mock objects created by the Mockery can be safely invoked from multiple threads. It's that easy. The Synchroniser has other features that help test multithreaded code, but more about those later.
The Synchroniser is currently in subversion HEAD and will be in the forthcoming jMock 2.6.0 release.